Develop a Blockchain Casino Game with Chainlink VRF
Developing a casino game on the blockchain requires more than just understanding game logic; it requires a method to generate provably fair randomness. Since blockchain networks are deterministic, generating random numbers that can't be tampered with is a challenge. This is where Chainlink VRF (Verifiable Random Function) comes in, providing secure and verifiable randomness that can be easily integrated into smart contracts. In this article, we will explore how to build a simple casino game using Chainlink VRF, complete with code snippets and explanations.
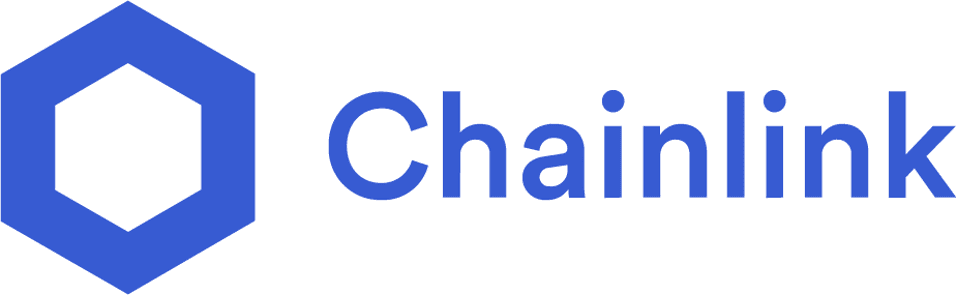
Overview of Chainlink VRF
Chainlink VRF is a decentralized oracle service that generates cryptographically secure random numbers. It works by producing random values alongside a cryptographic proof that verifies the integrity of these values. The proof is published and verified on-chain, ensuring that the randomness has not been tampered with by any party, including the oracle, contract developers, or users.
Why Use Chainlink VRF for a Casino Game?
Casino games rely heavily on randomness for fairness, especially for game elements like:
- Rolling dice.
- Drawing cards.
- Spinning roulette wheels.
Using Chainlink VRF ensures that the outcome of these actions is fair and cannot be manipulated by any participant or third-party. This builds trust with players, a crucial element for a successful blockchain-based casino.
Prerequisites
Before you start, make sure you have:
- A basic understanding of Solidity (the programming language for Ethereum smart contracts).
- MetaMask wallet set up and connected to a test network like Goerli.
- Some Goerli ETH and testnet LINK tokens to fund your VRF requests.
- Familiarity with Hardhat or another smart contract development environment.
Setting Up a Chainlink VRF Subscription
The first step in integrating Chainlink VRF is setting up a subscription:
- Create a Subscription: Visit the Chainlink VRF dashboard, select your desired test network (e.g., Goerli), and create a subscription account.
- Fund the Subscription: Fund your subscription with LINK tokens using the Chainlink faucet.
- Get Your Subscription ID: After creating and funding the subscription, copy the Subscription ID as you will need it for deploying your smart contract.
Writing the Casino Game Contract
For this example, we’ll build a simple dice game where the user bets on the outcome of a dice roll (a number between 1 and 6). If the user’s guess matches the random number generated, they win their bet amount times two.
Here’s the code for the casino game contract:
// SPDX-License-Identifier: MIT
pragma solidity ^ 0.8.7;
import "@chainlink/contracts/src/v0.8/interfaces/VRFCoordinatorV2Interface.sol";
import "@chainlink/contracts/src/v0.8/VRFConsumerBaseV2.sol";
contract CasinoGame is VRFConsumerBaseV2 {
VRFCoordinatorV2Interface COORDINATOR;
uint64 subscriptionId;
bytes32 keyHash;
uint32 callbackGasLimit = 100000;
uint16 requestConfirmations = 3;
uint32 numWords = 1;
uint256 public randomResult;
address public owner;
uint256 public betAmount;
address public player;
bool public betPlaced = false;
constructor(uint64 _subscriptionId, address _vrfCoordinator, bytes32 _keyHash)
VRFConsumerBaseV2(_vrfCoordinator)
{
COORDINATOR = VRFCoordinatorV2Interface(_vrfCoordinator);
subscriptionId = _subscriptionId;
keyHash = _keyHash;
owner = msg.sender;
}
// Player places a bet with a guess number
function placeBet(uint256 _betAmount, uint256 guess) external payable {
require(!betPlaced, "Bet already placed");
require(msg.value == _betAmount, "Bet amount must match the sent value");
require(guess >= 1 && guess <= 6, "Guess must be between 1 and 6");
betAmount = _betAmount;
player = msg.sender;
betPlaced = true;
requestRandomWords();
}
// Request random number from Chainlink VRF
function requestRandomWords() internal {
COORDINATOR.requestRandomWords(
keyHash,
subscriptionId,
requestConfirmations,
callbackGasLimit,
numWords
);
}
// Chainlink VRF callback function
function fulfillRandomWords(uint256, uint256[] memory randomWords) internal override {
randomResult = (randomWords[0] % 6) + 1; // Result between 1 and 6
checkBet();
}
// Check if player won or lost
function checkBet() internal {
if (randomResult == guess) {
// Player wins: double the bet amount
payable(player).transfer(betAmount * 2);
}
// Reset the game state
betPlaced = false;
player = address(0);
}
// Withdraw contract balance (for the owner)
function withdrawBalance() external {
require(msg.sender == owner, "Only owner can withdraw");
payable(owner).transfer(address(this).balance);
}
// Receive ETH
receive() external payable { }
}
Explanation of the Code
- Imports and Variables:
We import VRFConsumerBaseV2 to interact with the VRF and VRFCoordinatorV2Interface to access the Chainlink VRF functions.
Define subscriptionId, keyHash, and other VRF configuration values like callbackGasLimit and requestConfirmations.
Store the bet amount, player's address, and game state in variables.
- Constructor:
Initialize the VRFCoordinator with the subscriptionId and keyHash.
Set the contract's owner.
- Placing a Bet:
Players place bets using placeBet() by specifying a _betAmount and a guess (between 1 and 6).
The function ensures that the bet amount matches the sent ETH value and that only one bet can be active at a time.
Once the bet is validated, it calls requestRandomWords() to obtain a random number.
- Requesting Random Numbers:
requestRandomWords() uses the Chainlink VRF to generate randomness.
It specifies the subscription ID, gas limit, and other parameters for the randomness request.
- Handling Random Number Callback:
The fulfillRandomWords() function is called by Chainlink once randomness is generated.
It calculates a random number between 1 and 6 using randomWords[0] % 6 + 1, ensuring a dice-like outcome.
It then calls checkBet() to determine if the player's guess matches the random number.
- Checking the Bet:
If the player's guess matches the generated random number, they win, and their bet is doubled.
The contract then resets the game state, allowing new bets to be placed.
- Withdraw and Receive Functions:
The owner can withdraw the contract's balance using the withdrawBalance() function.
The receive() function allows the contract to accept ETH deposits directly.
Deploying the Contract
To deploy this contract:
- Set Up Environment:
Install the Chainlink contracts package using npm:
npm install @chainlink/contracts
- Deploy with Hardhat:
Write a deployment script specifying the VRFCoordinator address and other parameters.
Run the script to deploy the contract to the Goerli network.
- Add Consumer: Go back to the Chainlink VRF dashboard and add your deployed contract as a consumer to your subscription using its address.
Testing the Contract
- Place a Bet: Call the placeBet() function with a guess and bet amount. For example, if a player bets 0.01 ETH and guesses 3, they call:
await casinoGame.placeBet({ value: ethers.utils.parseEther("0.01") }, 3);
- Verify Randomness: The contract will automatically request a random number from Chainlink. Once the request is fulfilled, check the randomResult and determine if the player won or lost.
- Check Contract Balance: Ensure the payouts are correctly handled by checking the contract's balance before and after a win.